Javascript 4 - ES6
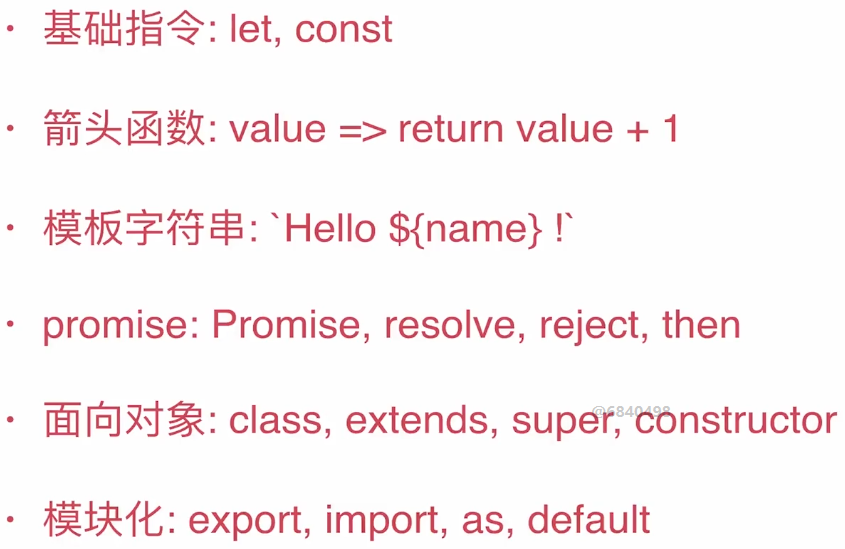
let/const block scope
let arr = [1,2,3,4]
for (let i = 0; i < arr.length; i++) {
}
console.log(i); // error
let arr = [1,2,3,4]
for (var i = 0; i < arr.length; i++) {
}
console.log(i); // 4
Hoisting
console.log(foo);
var foo = 1;
Arrow function
Arrow function no this, cannot be constructor, no prototype
// 函数声明
function test() {}
// 函数表达式
const test = function() {}
// 箭头函数
const test = () => {}
var obj = {
commonFn : function() {
console.log(this);
},
arrowFn : () => {
console.log(this);
}
}
obj.commonFn();
obj.arrowFn();
VM372:3 {commonFn: ƒ, arrowFn: ƒ}
VM372:6 Window {parent: Window, opener: null, top: Window, length: 2, frames: Window, …}
Template string
let getName = () => {
return 'test';
}
let str = `
<div>
<h1 class = "title">${getName()}</h1>
</div>
`;
document.querySelector('body').innerHTML = str;
object
var name = 'test',
age = 18;
var obj = {
name: name,
age: age,
getName: function() {
return this.name;
},
getAge: function() {
return this.age;
}
}
let name = 'test',
age = 18;
let obj = {
name,
age,
getName() {
return this.name;
},
['get' + 'Age']() {
return this.age;
}
}